How to use DeepSeek in offline mode
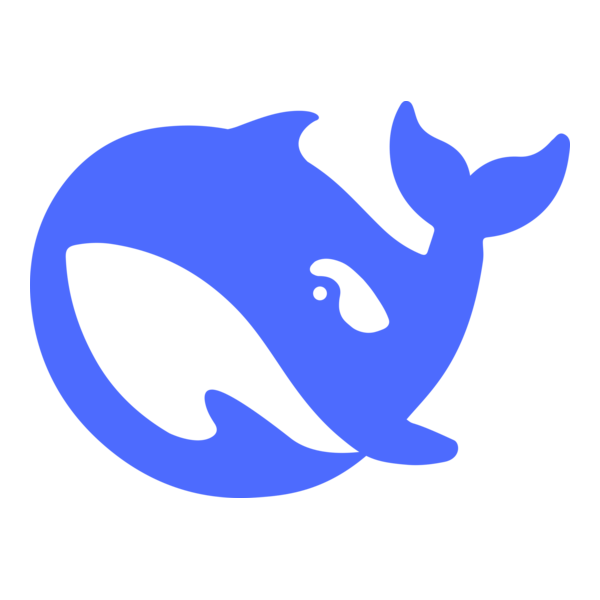
To use DeepSeek in offline mode, follow these steps:
1. Download the DeepSeek Model
- Visit the official DeepSeek website or repository (e.g., GitHub) to download the model files.
- Ensure you have the necessary dependencies and libraries installed on your system.
2. Set Up the Environment
- Install Python and required libraries (e.g., PyTorch, TensorFlow, or ONNX, depending on the model format).
- Use a virtual environment to manage dependencies:
bash
python -m venv deepseek-env
source deepseek-env/bin/activate # On Windows: deepseek-env\Scripts\activate
pip install -r requirements.txt # Install dependencies from the provided file
3. Load the Model Offline
- Place the downloaded model files in your project directory.
- Load the model in your Python script:
python
from transformers import AutoModelForCausalLM, AutoTokenizer
model_path = “path_to_downloaded_model”
tokenizer = AutoTokenizer.from_pretrained(model_path)
model = AutoModelForCausalLM.from_pretrained(model_path)
Example usage
input_text = “How does DeepSeek work?”
inputs = tokenizer(input_text, return_tensors=”pt”)
outputs = model.generate(**inputs)
print(tokenizer.decode(outputs[0], skip_special_tokens=True))
4. Run the Model
- Execute your script to use the model offline:
bash
python your_script.py
5. Optimize for Offline Use
- Ensure your system meets the hardware requirements (e.g., GPU for faster inference).
- Use quantization or smaller model variants if resources are limited.
6. Test and Validate
- Verify the model’s functionality by testing it with various inputs.
- Adjust parameters or fine-tune the model if necessary.
Notes:
- Offline mode requires all necessary files (e.g., tokenizers, configs) to be downloaded and accessible locally.
- Check the DeepSeek documentation for specific instructions or updates.